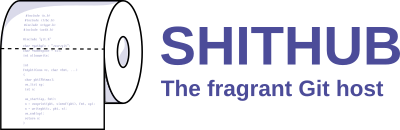
Shithub is a site for hosting git repositories. It is
running on git9,
hosted on 9front.
To get an account, or set up a group project email
Ori Bernstein <ori@eigenstate.org>.
To get started using shithub, read our
user guide
Shithub is a community service, written by people who
avoid browsers. As a result, the web interface is a work
in progress. Take a look at
what we want.
Repositories
- 9p.zone/infra
-
9p.zone server infrastructure
- 9p.zone/web
-
9p.zone web page
- aap/catvclock
-
Catclock based on original box art
- aap/jot
-
a very simple text editor based on lola's text.c
- aap/lola
-
an experimental window system
- aap/mlisp
-
A toy LISP inspired by MacLISP
- akw/sam
-
our beloved editor
- alex/8080
-
8080 emulator, disassembler, debugger
- alex/exif
-
Plan 9 tool for extracting EXIF data from JPEGs
- alex/img
-
Automatic image galleries using mk(1)
- alex/mntgen
-
a mntgen(4) for linux
- alex/mtk
-
Plan 9 support for MTK GPS data loggers
- alex/paste
-
basic pastebin cgi
- alex/powerware
-
Plan 9 support for PowerWare UPS
- alex/sitara
-
Plan 9 kernel for Ti Sitara SoC
- bebebeko/9p.zone
-
9p.zone
- bubstance/gridchat
-
9grid chat client
- cinap_lenrek/barrera
-
barrier mouse, keyboard and clipboard sharing client
- cinap_lenrek/blog
-
blog
- cinap_lenrek/rc
-
rc
- cinap_lenrek/repo
-
blog
- cinap_lenrek/stashfs
-
stashfs encrypted file store
- cinap_lenrek/trackerc
-
worst bittorrent tracker in rc
- covertusername/rd
-
windows rdp client for plan9/9front
- denzuko/9grid-releases
-
9grid/9p.zone scripts and releases (bleading edge on /n/disk/denzuko)
- denzuko/9pug-docs
-
Talks, slides, and Whitepapers for HPR, 9pug, 2600, and CDLUG
- denzuko/9pug-labs
-
Labs for HPR, 9pug, 2600hackers.com, and CDLUG
- denzuko/Nein-bugs
-
plan9/9front/p9p/planD/Golang quarks and bugs
- dippywood/9intro
-
9Intro
- echoline/drawterm
-
drawterm patches
- echoline/monome
-
plan 9 monome programs
- echoline/tpi
-
turing pi shit
- echoline/util
-
utilities
- edk/iwp9-proceedings
-
Proceedings of IWP9
- ethandl/nime
-
A Japanese IME for Plan 9
- fulton/9scripts
-
my rc scripts
- fulton/emailconfig
-
Unnamed repository; edit this file 'description' to name the repository.
- fulton/namespace-example
-
Unnamed repository; edit this file 'description' to name the repository.
- fulton/privategrid
-
A private grid based off the public grid
- g_w1/libdraw-zig
-
a zig client library for plan 9's draw protocol
- gab/mytestrepo
-
my test repo
- gab/test
-
test
- garden/clone
-
Fast parallel file copy for Plan 9
- garden/esig
-
extended sig
- garden/logrotate
-
A mutli-generation, multi-logfile rotator
- garden/shithub
-
this site
- garden/touchui
-
Plan 9 Touch UI Ideas
- garden/vt
-
you got my vt in my st
- garden/werc
-
a minimalist document management system
(converted from the canonical mercurial repo at https://code.9front.org/hg/werc)
- garden/wiki.9front.org
-
9front public wiki
- grobe0ba/9ficl
-
a port of the FICL forth system used in the freebsd and illumos bootloaders to 9
- grobe0ba/freetype+ttf2subf
-
the latest version of freetype with ttf2subf, all for 9front
- grobe0ba/gridchat
-
my modified gridchat client
- grobe0ba/sandbox
-
modified service files and scripts to allow sandboxing of incoming cpu connections on 9front
- grobe0ba/tarsum
-
a small lua tool to update the checksum of a tar file so you can do horrible things with it
- grobe0ba/tcp80
-
the enterprise edition of tcp80, supporting integrated execfs, multiple hostname support, error page redirection, and Content-Type headers
- grobe0ba/tlsclient
-
A fork of moody's tlsclient with vendored boringssl
- grobe0ba/werc
-
a minimalist document management system
(converted from the canonical mercurial repo at https://code.9front.org/hg/werc)
- henesy/binrc
-
bin/rc
- henesy/cursedfs
-
WIP fs shim for ncurses
- henesy/fuzz
-
Toy fuzzer for Plan 9 syscalls
- henesy/kandr
-
K&R exercises
- henesy/libds
-
toy data structures library
- henesy/libmujs
-
WIP libmujs port to 9front
- henesy/limbobyexample
-
Examples for the Limbo Programming Language
- henesy/olednews
-
MNT Reform 2 OLED text scroller
- henesy/oledsaver
-
MNT Reform 2 OLED bit art screensavers
- henesy/purgatorio
-
Now I shall sing the second kingdom there where the soul of man is cleansed, made worthy to ascend to Heaven.
- hexyl/alternative
-
http
- hexyl/ditto
-
tiny programming language
- hexyl/hexal
-
probably some code
- hexyl/libtroll
-
probably some code
- igor/clock
-
another kind of clock
- igor/hugo
-
9front port of https://gohugo.io
- igor/tcp80
-
yet another variant of tcp80 (see https://9lab.org/plan9/web-server-with-go-based-static-site-generator-hugo/)
- igor/tlssrv.sni
-
tlssrv(8) with Server Name Indication (SNI) support
- igor/volume
-
volume control
- jdrm/9utils
-
Scripts and utils
- jdrm/ddate
-
Discordian calendar
- jgstratt/acme-themes
-
acme, but it uses rio-themes themes if available.
- jgstratt/klondike
-
Klondike for Plan 9.
- josuah/dmenu
-
dynamic line selection menu
- jrsharp/heymac-node
-
A HeyMac implementation for ESP32-based LoRa boards
- julienxx/castor9
-
A gemini browser
- julienxx/finge.rc
-
A minimal finger server
- julienxx/masto9
-
a mastodon client
- julienxx/toe
-
A finger clone
- k0ga/equis
-
X11 port to plan9
- k0ga/scc
-
simple c99 compiler
- k0ga/st
-
St port for plan9
- kemal/ircs
-
fork of jpms http://plan9.fi/src/ircs.tgz
- kitzman/chessfs
-
chessfs(4), a chess game written in Go
- kitzman/dddb
-
WIP RDBMS in Limbo
- kitzman/devlimit-patch
-
system limits for 9front
- kitzman/p9-stm32-example-bare
-
plan9 stm32 bare-metal example
- kitzman/p9-stm32-example-os
-
WIP rtos serving 9P via uart
- kitzman/rc-nntpd
-
NNTP server written in rc
- kitzman/scripts
-
rc and lua scripts, patches, themes, etc
- kitzman/stm32up
-
stm32 flashing utility
- kitzman/utotp
-
factotum totp utility
- kvik/attrdb.lua
-
Attribute database inspired by Inferno's attrdb(2)
- kvik/bin.rc
-
/usr/kvik/bin/rc
- kvik/clone
-
Fast parallel file copy for Plan 9
- kvik/docs.9front.org
-
Knowledge front
- kvik/ham
-
Sam but Ham
- kvik/lu9
-
Lua standalone interpreter for Plan 9
- kvik/lu9-lpeg
-
LPeg library for lu9
- kvik/lu9-lua
-
Native (not APE) port of liblua to Plan 9
- kvik/lu9-p9
-
Lua module providing a Plan 9 system interface
- kvik/mousetrap
-
Mouse event filter
- kvik/mq
-
message queue
- kvik/plumbreport
-
Report plumber activity
- kvik/post
-
publish a file descriptor
- kvik/rngfs
-
Random instructional 9p file server
- kvik/tcp80
-
Cinap's tcp80, with modifications
- kvik/treepack
-
File tree marshaling
- kvik/ugh
-
Frugal website generator
- kvik/union
-
Recursive union builder
- kvik/unionfs
-
Deep union file server for Plan 9
- kvik/watch
-
Run command on file change, for Plan 9
- kvik/x
-
Plan 9 tools for dealing with UNIX®
- kws/elf-loader
-
probably some code
- kws/loong
-
LoongArch kernel
- kws/newlib
-
Newlib userspace
- kws/weiqi
-
probably some code
- kws/wip
-
probably some code
- launchpad/test
-
test repo
- libreboot/libmk
-
libreboot build system
- libreboot/libwww
-
libreboot website
- libreboot/libwww-img
-
images for use with lbwww
- maht/repo
-
Maht's dumping ground
- mia/n900
-
nokia n900 plan 9 kernel
- moody/atom
-
atom feed generator
- moody/blake2
-
blake2 9front port
- moody/build
-
tools for build engine games
- moody/candycrisis
-
Candy Crisis Port
- moody/choc
-
very wip chocalate doom port
- moody/cstory
-
cave story
- moody/dfc
-
data field compiler
- moody/duke3d
-
duke3d port
- moody/fossil
-
fossil imported from 9legacy
- moody/gbaex
-
game boy advance examples
- moody/hammer
-
some kind of language
- moody/heretic
-
heretic port
- moody/hexen
-
hexen port
- moody/hmap
-
over engineered hash map
- moody/ips
-
IPS patcher
- moody/iso
-
nightly iso buildbox
- moody/kdict
-
kanji lookup by radical
- moody/mpl
-
old music player
- moody/neatmkfn
-
neatmkfn port
- moody/neatpost
-
neatpost port
- moody/neatroff
-
neatroff port
- moody/paste
-
paste scripts
- moody/pokecrystal
-
build pokemon crystal using mk
- moody/pokered
-
build pokemon red using mk
- moody/powernv
-
power9 kernel
- moody/pse
-
pokemon save editor
- moody/raven
-
tools for ravensoft doom clones
- moody/rc-gemd
-
rc gemini server
- moody/rewise
-
probably some code
- moody/rgbds
-
rgbds port
- moody/riscv
-
risc-v 9front port
- moody/rott
-
wip rott port
- moody/seedbox
-
A program to manage swaths of torrents
- moody/stats
-
adaptive stats
- moody/tinygl
-
tinygl plan9 port
- moody/tlsclient
-
probably some code
- moody/wipeout
-
wipeout port
- moody/zelda3
-
zelda 3: A link to the past 9front port
- nikita/acme
-
Acme with some personal touches
- nikita/acme-scrolling
-
Acme patch that adds Rio-like scrolling
- nikita/align
-
basic term text alignment tool
- nikita/clear
-
clears the terminal without affecting state
- nikita/hl
-
Simple text highlighter, used with patched lola
- nikita/lola
-
Tinkering with aap's window system. Adds text highlighting.
- nikita/plumb-complete
-
experiment with plumbing completions
- nikita/rc
-
patched rc with custom prompt
- nikita/rcmisc
-
my rc scripts etc
- nikita/rio
-
rio with some personal touches
- nikita/rioc
-
rio with support for colored text
- nikita/windir
-
terminal widget that reports the directory contents of the current window
- ori/5v
-
5v memory verifier
- ori/9bench
-
benchmarks for 9front
- ori/Nail
-
A rewrite of Acme mail
- ori/Slide
-
slide show
- ori/acmed
-
acme cert client
- ori/diff
-
diff with merge3
- ori/gefix
-
tool to fix up broken gefs
- ori/getest
-
misc test scripts for gefs
- ori/git9
-
probably some code
- ori/hg-archive
-
probably some code
- ori/libdraw.myr
-
Unnamed repository; edit this file 'description' to name the repository.
- ori/mc
-
myrddin compiler
- ori/mkarchive
-
mlmmj web archive
- ori/mq
-
mq rewrite
- ori/pki
-
pki implementation
- ori/regress
-
probably some code
- ori/shithub
-
this site
- ori/tcp80
-
tcp80: my version
- ori/test
-
test
- ph/misc
-
various stuff
- ph/mycel
-
Rudimentary web browser with HTML5/CSS support
- ph/wildlife
-
Plan 9 port of MS Dangerous Creatures
- ph/xui
-
simple ui lib, very wip
- phil9/calfs
-
'a 9p calendar fs'
- phil9/candlestick
-
'a candlestick chart widget'
- phil9/fm
-
a fuzzy matcher selection gui
- phil9/gj
-
greppable json
- phil9/gopher
-
a gopher browser for 9front
- phil9/graphical-algorithms
-
'some graphical experiments with slug'
- phil9/grapple
-
graphical plumbable lines
- phil9/ifilter
-
image color filters
- phil9/lua9
-
an lua interpreter with plan9 bindings for 9front
- phil9/mongrel
-
'a mail reader for 9front'
- phil9/nc
-
'nein-commander: a dual pane file manager for plan9'
- phil9/nordle
-
'a Wordle game clone'
- phil9/rrss
-
convert rss feeds to werc barf format
- phil9/shithub
-
shithub website
- phil9/slug
-
'9front visual programming'
- phil9/spit
-
'a simple presentation tool'
- phil9/svg
-
a native svg image viewer for plan9
- phil9/tcp80x
-
an http server with some basic CGI support
- phil9/vcrop
-
a visual image cropper for 9front
- phil9/vdict
-
'a visual DICT client'
- phil9/vdiff
-
a git/diff output viewer
- phil9/vdir
-
a visual directory browser for 9front
- phil9/vexed
-
'an hex editor'
- phil9/view
-
an image viewer
- phil9/vim
-
a plan9 port of the vim editor
- phil9/vshot
-
visual screenshotter for plan9
- pix/neoventi
-
neoventi: venti, but neo
- pmikkelsen/apl10
-
Yet another attempt at writing an APL interpreter for Plan 9
- pmikkelsen/gitonline
-
A git repo browser for rc-httpd
- pmikkelsen/guifs
-
Experimental filesystem for creating GUI applications
- pmikkelsen/lpa
-
LPA: experimental APL interpreter
- pmikkelsen/neinchat
-
Some chat server over 9p
- pmikkelsen/pprolog
-
Prolog on Plan 9
- qbit/exie
-
exie configs
- qbit/gover
-
gotip for releases
- qbit/ninethings
-
some stuff, maybe some things
- qwx/3d
-
useless graphics tryouts
- qwx/alienpatch
-
Patches for alien software
- qwx/asif
-
Algorithm tryouts, barren
- qwx/audio-stretch
-
time domain harmonic scaling, npe port
- qwx/city
-
city building, wip
- qwx/dmap
-
Doom map viewer, wip
- qwx/dporg
-
doomrpg reimplementation
- qwx/fork
-
there is no fork
- qwx/fplay
-
pplay with frequency domain visualization, pending merge
- qwx/ft²
-
fork of sigrid's fork of ft2-clone
- qwx/gm4s
-
we deserve a better tetromino stacker than 4s
- qwx/libopusenc
-
opus encoder lib
- qwx/misc
-
misc bullshit
- qwx/mkey
-
Software MIDI piano
- qwx/mst
-
MIDI typesetter or sexually transmitted disease, au choix
- qwx/omidi
-
Standalone midi player port with opl2 emulation
- qwx/opl2
-
IMF interpreter and OPL2-only emulator
- qwx/opus
-
opus fork
- qwx/opus-tools
-
opus decoder/encoder
- qwx/opusfile
-
opus decoder lib
- qwx/patch
-
Misc. 9front patches
- qwx/pcx
-
PCX decoder
- qwx/peu
-
Misc. Doom editing utilities, most are elsewhere
- qwx/pico
-
Plan 9 awk implementation of the pico image compositing language, wip
- qwx/pplay
-
Visual PCM audio player
- qwx/prez
-
prezbo's paint(1) for notes and presentations
- qwx/qk1
-
Quake 1 and quakeworld ports
- qwx/qk2
-
Quake 2 + mission packs + crbot ports
- qwx/qk3
-
Quake 3 port, very wip
- qwx/rc
-
Misc. rc scripts
- qwx/sce
-
Starcraft Brood War engine reimplementation, very wip
- qwx/sf2mid
-
midi player using sf2 sound banks
- qwx/sm2
-
Supermemo2-assisted spaced repetition memorization + old examples
- qwx/syro
-
syro encoder for korg volca sample 2
- qwx/tbs
-
Turn-based board game engine, wip
- qwx/u6m
-
Ultima 6 m format audio decoder
- qwx/wavenc
-
quick dr_libs wavenc port for ft2 pending a simpler and better one
- qwx/weu
-
Wolfenstein 3D editing utilities, wip
- qwx/wl3d
-
Wolfenstein 3D reimplementation
- rminnich/nix
-
nix kernel
- rodri/3dee
-
3D Environments in Plan 9
- rodri/amd64-simd
-
SIMD trials
- rodri/ballistics
-
External ballistics simulator
- rodri/battleship
-
Multi-user on-line Sink the Fleet
- rodri/boids
-
Bird flock simulator
- rodri/brokentoys
-
A Toy Story
- rodri/catphone
-
VoIP softphone worth a cat
- rodri/etoys
-
Software Toys
- rodri/imagetools
-
image processing tools for plan 9
- rodri/libgraphics
-
3D computer graphics library
- rodri/libobj
-
OBJ parser
- rodri/libstl
-
STL parser
- rodri/mkweb
-
rodri's tiny web framework
- rodri/musw
-
multi-user Spacewar!
- rodri/rcfitness
-
Rc tools for fitness tracking
- rodri/renderfs
-
3D rendering server
- rodri/rhoc
-
hoc but rhoc
- rodri/semblance
-
A wannabe shading language
- rodri/threadpool
-
parallel experiments lain
- rodri/tinyrend
-
Tiny renderer lessons and experiments
- rodri/volume
-
volume control knob for plan 9
- rodri/wpq
-
Wikipedia query tool
- romi/9front-config
-
9front Configuration
- romi/ktrans
-
archived
- rosie/9volt
-
Simple RC Revolt client
- rvs/tinyemu
-
tinyemy port for Plan9
- sciso/bez
-
half a toy project
- shepard/dormer
-
library for a game
- shepard/test
-
test
- shepard/tree
-
tree command
- sigrid/9pro
-
Plan 9-related tools for Unix-like operating systems.
- sigrid/aacdec
-
audio/aacdec for Plan 9
- sigrid/aacenc
-
audio/aacenc for Plan 9
- sigrid/atlas
-
Creates an atlas texture out of smaller images and dumps as Plan 9 image and mapping between those.
- sigrid/aubio
-
A library for audio and music analysis, port to Plan 9
- sigrid/bar
-
(ships with 9front) A small bar with battery charge, date and time, for Plan 9.
- sigrid/bench9
-
Benchmarking for 9.
- sigrid/c9
-
Low-level 9p client and server in C.
- sigrid/cflood
-
"Color Flood" game clone for Plan 9.
- sigrid/dav1d
-
AV1 decoder library port for 9.
- sigrid/dumb
-
audio/moddec: module music decoder for Plan 9
- sigrid/evdump
-
(ships with 9front) Report input events on 9front.
- sigrid/ext4srv
-
(ships with 9front) Read/write file server for Ext2, Ext3 and Ext4 file systems with journaling and extents support.
- sigrid/flite
-
Text to speech for Plan 9, port of flite
- sigrid/fnt
-
Font loading, rendering and text shaping engine for 9front.
- sigrid/fontsel
-
(ships with 9front) Font selector for Plan 9.
- sigrid/ft2-clone
-
FastTracker II clone port for Plan 9
- sigrid/ft2play
-
audio/ft2dec for Plan 9. Decodes XM/MOD/FT.
- sigrid/gemnine
-
Gemini browser for Plan 9.
- sigrid/h264bsd
-
H264 baseline decoder library for Plan 9.
- sigrid/hantro9
-
Hantro G1/G2 VPU tools for 9front
- sigrid/hj264
-
H.264 video capture & encoding for Plan 9
- sigrid/hx
-
A faster version of "hexdump -C".
- sigrid/imgtools
-
Some random image-related tools.
- sigrid/itdec
-
ImpulseTracker module decoder.
- sigrid/jacksense
-
A small program to track whether headphones are connected to jack or not, and switch between speaker and headphones output accordingly.
- sigrid/jbig2
-
JBIG2 decoder for Plan 9.
- sigrid/jp2
-
JPEG2000 decoder for Plan 9.
- sigrid/jxl
-
JPEG XL decoder for Plan 9
- sigrid/leaf
-
Lightweight Embedded Audio Framework
- sigrid/libsamplerate
-
Port of libsamplerate to Plan 9.
- sigrid/libtags
-
(ships with 9front) A cross-platform library for reading tags, designed for highly constrained environments.
- sigrid/libvpx
-
libvpx (vp8/vp9 library) for Plan 9
- sigrid/lwext4
-
(ships with 9front) Library for Ext2/Ext3/Ext4 file system operations.
- sigrid/mcfs
-
A tool for working with media container formats.
- sigrid/microui
-
A tiny, portable, immediate-mode UI library written in ANSI C. Plan 9 version.
- sigrid/minivmac
-
A 9front port of minivmac, an emulator of classical Mac computers.
- sigrid/mkfaces
-
Some kind of Gravatar `face(6)` fetcher for Plan 9?
- sigrid/mp3dec
-
A (better) drop-in replacement for audio/mp3dec.
- sigrid/neindaw
-
Some kind of DAW for 9front? An ongoing effort.
- sigrid/npe
-
Native Porting Environment for Plan 9
- sigrid/nvi
-
Download a youtube video on Plan 9
- sigrid/openh264
-
openh264 port to 9front
- sigrid/orca
-
Plan 9 port of ORCΛ, an esoteric programming language-sequencer for music.
- sigrid/pc
-
Programmer's calculator pc(1) ported to Unix-like OS
- sigrid/pdffs
-
PDF as a file system. WIP
- sigrid/picker
-
HSLuv, HPLuv, and RGB color picker for Plan 9.
- sigrid/pitch
-
A simple voice pitch analyzer.
- sigrid/pt2-clone
-
ProTracker 2 clone, ported to Plan 9
- sigrid/qoistream
-
QOI livestreamer
- sigrid/riow
-
(ships with 9front) Some kind of window management experiments with rio.
- sigrid/rtmp
-
RTMP client for Plan 9
- sigrid/snippets
-
Various code snippets and scripts for 9front.
- sigrid/soundpipe
-
SoundPipe port to 9front (npe)
- sigrid/sox
-
SoX, Swiss Army knife of sound processing. A port to 9front
- sigrid/tocursor
-
Converts a Plan 9 image to Cursor C structure.
- sigrid/treason
-
A video player for 9front.
- sigrid/xmpp
-
XMPP client for Plan 9.
- sigrid/zuke
-
(ships with 9front) A music player for Plan 9.
- sirjofri/arcs
-
scratch hologram gcode generator
- sirjofri/blie
-
blended layer image editor
- sirjofri/drawfs
-
persistent drawfs client
- sirjofri/drawterm-fdroid
-
android drawterm for fdroid
- sirjofri/eesp
-
eenter snarf/paste
- sirjofri/epublish
-
e-publishing tools
- sirjofri/fingerd
-
plan 9 finger server
- sirjofri/fractals
-
fractals
- sirjofri/gpufs
-
gpufs and spirvd
- sirjofri/gpufswip
-
gpu filesystem iwp9
- sirjofri/gridirc
-
gridchat irc wrapper
- sirjofri/libgit
-
git C library
- sirjofri/libini
-
parse ini files
- sirjofri/libnate
-
UI framework inspired by Slate
- sirjofri/livedoc
-
live plumb troff documents
- sirjofri/map
-
open street map for plan 9
- sirjofri/mapfs
-
map filesystem for OSM tiles
- sirjofri/news2atom
-
news2atom
- sirjofri/notif
-
notification system built around plumber
- sirjofri/nstats
-
stats for console output
- sirjofri/plumbto
-
gui plumb selector
- sirjofri/pronterfs
-
3d printer filesystem
- sirjofri/puzzles
-
port of simon tatham puzzle collection
- sirjofri/rssfill
-
rssfill
- sirjofri/sergui
-
(somewhat) serious guide to 9front
- sirjofri/sirjofri_de
-
sirjofri.de
- sirjofri/spirva
-
spirv assembler
- sirjofri/spread
-
spreadsheet editor
- sirjofri/todofs
-
todo filesystem
- sirjofri/totp
-
totp client
- sirjofri/touchuichal
-
paper touch ui challenges
- sirjofri/vcardfs
-
vcard filesystem for plan 9
- sirjofri/webbox
-
expose trusted web companion
- sirjofri/winview
-
visual winwatch
- sirjofri/xml-9atom
-
xml tools from 9atom
- sirjofri/xmltools
-
xml tools
- sirjofri/zet
-
Zettelkasten for acme
- sl/1f300
-
1F300, by Stanley Lieber
- sl/1oct1993
-
1OCT1993, by Stanley Lieber
- sl/300c300d
-
300C300D, by Stanley Lieber
- sl/9bbs
-
citadel style bbs for plan 9, written in rc
- sl/barf
-
werc app (blog, image, etc.)
- sl/bmothra
-
sl's fork of stock 9front mothra.
- sl/maude_mold
-
Maude Mold, by Stanley Lieber
- sl/no_memory
-
no_memory, by Stanley Lieber
- sl/reverse_crime
-
REVERSE CRIME, by Stanley Lieber
- sl/rrss
-
rrss, trrss - RSS feed readers
- sl/thrice_great_hermes
-
Thrice Great Hermes, by Stanley Lieber
- sl/werc
-
A sane web anti-framework
- slashscreen/ricket
-
A WASM runtime for plan 9
- smj/bootcamp
-
SDF Plan9 BootCamp
- smj/figlet
-
figlet - text banner generator of a variety of typefaces
- smj/rc
-
various rc scripts
- smj/schnek
-
schnek - chase down decaying food before you starve.
- spew/MicroHs
-
Porting MicroHs
- spew/aplay
-
audio player
- spew/libscroll
-
General purpose scrolling
- spew/mez
-
IRC Client for Soju Bouncer
- spew/sl
-
fork of sl for development
- spew/squint
-
Squinting at power series for 9front
- tevo/cc65-plan9
-
Port of the cc65 65(C)02 development toolchain
- tevo/cuefs
-
Plan 9 filesystem for mounting cuesheets as a set of separate files
- tevo/diskcheck
-
Incomplete badblocks workalike for Plan 9
- tevo/doll
-
Plan 9 a.out → DOL converter
- tevo/pointless
-
Small static website generator
- tevo/prismriver
-
(Skeleton for) a Plan 9 audio synthesis library
- tevo/waffle
-
Simple but flexible gopher server for Plan 9
- tevo/widget
-
Small widget library for Plan 9
- thedaemon/dpaint
-
fork of paint(1)
- thedaemon/rc
-
rc scripts
- unobe/drawterm
-
9front drawterm with some OS X improvements
- unobe/libdvdcss
-
WIP Plan9 port of libdvdcss
- unobe/patches
-
patches, both good and bad
- unobe/x
-
Plan 9 tools for dealing with UNIX® (based off of kvik/x)
- yk/fontsrv
-
file system access to scalable fonts ported from plan9port
- yk/rd
-
Remote Desktop Protocol client
- zgasma/desereter
-
Tool to convert English to the Deseret Alphabet
- zgasma/martian9
-
WIP - simple scheme interpreter in base OCaml